I know you are trying to help and I appreciate your time an effort. Do not think I am trying to be a freak or something.
Zero is OrderNum that you supply in E9 and it will generate next OrderNum on Save of a header. That’s also what Epicor says when I am trying to enter the next OrderNum:
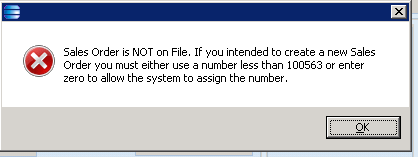
And I guess you mentioned that I need to create a Header first and then use a valid OrderNum to from that Header to create detail for that Header and that’s what I am doing. Let me share the latest code here:
SalesOrderDataSetType ds = new SalesOrderDataSetType();
//Populating Header fealds
SalesOrderDataSetTypeOrderHed soDSHed = new SalesOrderDataSetTypeOrderHed();
ds = soService.GetNewOrderHed("TEST", ds, callContextIn, out callContextOut);
soDSHed.Company = "TEST";
soDSHed.OrderNum = 0;
soDSHed.CustNum = 1;
soDSHed.CustomerCustID = "TEST";
soDSHed.ShipViaCode = "UPSG";
ds.SalesOrderDataSet[0] = soDSHed;
soService.SubmitNewOrder("TEST", ds, callContextIn, out callContextOut);//This will create only a header. After this call new order with OrderNum and other info already in Epicor.
soService.OnChangeofSoldToCreditCheck("TEST", 0, "TESTCUST", ds, callContextIn, out string creditLimit, out bool cont, out callContextOut);
//soService.ChangeSoldToID("TEST", ds, callContextIn, out callContextOut); you can comment this one as it will return a warning that Header did not changed
//soService.ChangeCustomer("TEST", ds, callContextIn, out callContextOut); you can comment this one as it will return a warning that Header did not changed
soService.MasterUpdate("TEST", checkForResponse, "OrderHed", 1, 143991, weLicensed, ds, callContextIn, out weLicensed, out string RespMsg, out string DispMasg, out string complMsg, out string tt, out callContextOut);
var list = soService.GetList("TEST", "OrderNum=143991", 100, 0, callContextIn, out bool morePages, out callContextOut); // Where clause is OrderNum=143991 is for just retrieve the latest one and not all of them for now.
//Grabbing an OrderNum value
OrderHedListDataSetTypeOrderHedList listDataSet = new OrderHedListDataSetTypeOrderHedList();
int validOrderNum = 0;
foreach (var item in list.OrderHedListDataSet)
{
if (item is OrderHedListDataSetTypeOrderHedList)
{
listDataSet = (OrderHedListDataSetTypeOrderHedList)item;
validOrderNum = listDataSet.OrderNum;
}
Trace.WriteLine("Line 9 -----------------" + listDataSet.OrderNum);
}
//Populating Detail fields
SalesOrderDataSetTypeOrderDtl soDSDtl = new SalesOrderDataSetTypeOrderDtl();
ds = soService.GetNewOrderDtl("TEST", ds, validOrderNum, callContextIn, out callContextOut);
soDSDtl.Company = "TEST";
soDSDtl.OrderNum = validOrderNum;
soDSDtl.OrderLine = 1;
soDSDtl.PartNum = "6.WD8";
soDSDtl.CustNum = 1;
soDSDtl.SellingQuantity = 1;
soDSDtl.LineType = "PART";
string partNum = soDSDtl.PartNum;
bool SubPartExists = false;
bool isPhantom = false;
string uomCode = "";
Trace.WriteLine("Line 2 -----------------");
soService.ChangePartNumMaster("TEST", ref partNum, ref SubPartExists, ref isPhantom, ref uomCode, "", "", false, false, false, true, true, true, ds, callContextIn, out string cDeleteComp, out string questStr, out string cWarningMess,out bool multyMatch, out bool promptToExpBOM, out string cConfigPart, out string cSubPart, out string explBOM, out string cMsgType, out bool multiSubsAvail, out bool runOutQtyAvail, out callContextOut);
Trace.WriteLine("Line 3 -----------------");
soService.ChangeSellingQtyMaster("TEST", ds, soDSDtl.SellingQuantity, false, false, true, true, false, true, partNum, "","","","EA", 1.0m,callContextIn, out string pcMess, out string pcNeqQty,out string opWarning, out string cSellingQuantity, out callContextOut);
Trace.WriteLine("Line 4 -----------------");
ds.SalesOrderDataSet[1] = soDSDtl;
Trace.WriteLine("Line 5 -----------------");
soService.MasterUpdate("TEST", checkForResponse, "OrderDtl", 1, validOrderNum, weLicensed, ds, callContextIn, out weLicensed, out string RespMsgDtl, out string DispMasgDtl, out string complMsgDtl, out string ttDtl, out callContextOut);
Trace.WriteLine("Line 6 -----------------");
Methods calls occurs in a proper order like TraceLog shows. Except for SubmitNewOrder.
Let me know if you need more info.